Exploring servers and applications, I see NestJS as a top choice for building efficient servers with TypeScript. This article aims to help you understand database integration and authentication in NestJS. We’ll cover NestJS basics, database options, and how to use TypeORM for database connections.
We’ll also show you how to set up JWT for secure authentication. This will equip you with the knowledge to handle these key topics smoothly.
Introduction to Database Integration in NestJS
Database integration is essential for any web application, and NestJS provides out-of-the-box solutions to connect to databases easily. Whether you are using SQL (like MySQL, PostgreSQL) or NoSQL (like MongoDB), NestJS supports various database types via TypeORM and Mongoose for object-relational mapping (ORM).
Setting Up a Database in NestJS
For database integration, the popular ORM for SQL databases is TypeORM, and for MongoDB, Mongoose is often used. Let’s walk through the steps of setting up an SQL database like MySQL in NestJS using TypeORM.
Step 1: Install TypeORM and MySQL Packages
npm install @nestjs/typeorm typeorm mysql2
Step 2: Configure TypeORM in Your App Module
In app.module.ts
, you need to configure the TypeORM module by adding your database credentials.
import { Module } from '@nestjs/common'; import { TypeOrmModule } from '@nestjs/typeorm'; @Module({ imports: [ TypeOrmModule.forRoot({ type: 'mysql', host: 'localhost', port: 3306, username: 'root', password: 'password', database: 'nestjs_db', entities: [__dirname + '/../**/*.entity{.ts,.js}'], synchronize: true, }), ], }) export class AppModule {}
Step 3: Create an Entity
Next, create an entity that represents the database table. For example, let’s create a User
entity.
import { Entity, Column, PrimaryGeneratedColumn } from 'typeorm'; @Entity() export class User { @PrimaryGeneratedColumn() id: number; @Column() name: string; @Column() email: string; @Column() password: string; }
Authentication in NestJS
When building a NestJS app, picking the right authentication strategy is key. I can use JSON Web Tokens (JWT), OAuth, or local methods to keep user data safe. Passport NestJS is a top choice for smoothly integrating these strategies. It makes adding security to my app easier.
Strategies for Authentication
There are many ways to secure an app. Each method has its own strengths and fits different needs. For example:
- JWT Tokens: Great for apps that don’t store user data.
- OAuth: Allows users to log in with services like Facebook without sharing passwords.
- Local Strategies: Uses traditional login forms with usernames and passwords.
My choice depends on what my app needs and how users will interact with it.
Step 1: Install Passport and JWT Packages
npm install @nestjs/jwt @nestjs/passport passport passport-jwt
Step 2: Configure JWT Module
In auth.module.ts
, import the JwtModule and configure it with your secret key.
import { Module } from '@nestjs/common'; import { JwtModule } from '@nestjs/jwt'; @Module({ imports: [ JwtModule.register({ secret: 'yourSecretKey', signOptions: { expiresIn: '1h' }, }), ], }) export class AuthModule {}
Step 3: Implement Authentication Logic
Next, we will implement authentication logic by creating a service and controller to handle user registration and login.
Auth Service (auth.service.ts
)
import { Injectable } from '@nestjs/common'; import { JwtService } from '@nestjs/jwt'; @Injectable() export class AuthService { constructor(private readonly jwtService: JwtService) {} async validateUser(username: string, pass: string): Promise<any> { // Validate user by checking in the database (dummy implementation) const user = { username: 'test', password: 'test' }; if (user && user.password === pass) { return { userId: 1, username: user.username }; } return null; } async login(user: any) { const payload = { username: user.username, sub: user.userId }; return { access_token: this.jwtService.sign(payload), }; } }
Auth Controller (auth.controller.ts
)
import { Controller, Post, Body } from '@nestjs/common'; import { AuthService } from './auth.service'; @Controller('auth') export class AuthController { constructor(private readonly authService: AuthService) {} @Post('login') async login(@Body() body) { const user = await this.authService.validateUser(body.username, body.password); if (!user) { return 'Invalid credentials'; } return this.authService.login(user); } }
Database Integration and Authentication Example
Let’s combine both database integration and authentication in a practical example. Suppose we want users to register and log in to the application, storing user details in the MySQL database.
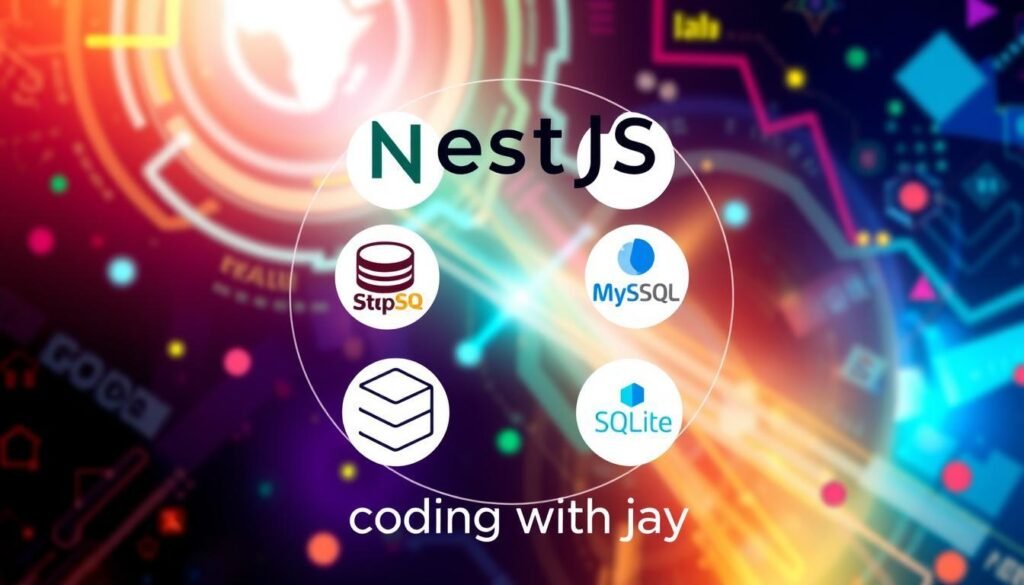
Step 1: Register the User in Database
We first create an API endpoint that allows users to register by saving their details (name, email, password) in the database.
import { Controller, Post, Body } from '@nestjs/common'; import { UserService } from './user.service'; @Controller('users') export class UserController { constructor(private readonly userService: UserService) {} @Post('register') async register(@Body() createUserDto: any) { return this.userService.create(createUserDto); } } import { Injectable } from '@nestjs/common'; import { InjectRepository } from '@nestjs/typeorm'; import { Repository } from 'typeorm'; import { User } from './user.entity'; @Injectable() export class UserService { constructor( @InjectRepository(User) private usersRepository: Repository<User>, ) {} create(user: any) { return this.usersRepository.save(user); } }
Step 2: Secure Login with JWT
When a user logs in, you’ll verify the credentials, generate a JWT token, and send it back for future authenticated requests.
This post covered the essential steps to integrate a database and implement authentication in a NestJS application. You can build robust and scalable server-side applications by using TypeORM for seamless database management and JWT for secure authentication. We demonstrated setting up MySQL with TypeORM, creating user entities, and securing login functionality using Passport and JWT in NestJS.
Conclusion
NestJS simplifies the process of database integration and authentication, making it a top choice for building efficient web applications. With proper implementation, you can ensure security, scalability, and maintainability. Start building your secure NestJS application today by leveraging these powerful tools!